JSON stands for Javascript Object Notation and it is a lightweight format for storing text-based information. It can easily be read and written by humans and it has no functionality on its own in addition to simply storing information in a platform-independent way.
Originally JSON was developed to be used in Javascript but currently most (if not all) computer languages have some sort of support for it natively. Lately even database engines, for example, MySQL 8.0 added support for dynamic JSON-based columns and provide SQL extensions to easily query data inside them.
JSON data types
JSON is strongly typed (as opposed to, for example, XML, which is weakly typed), meaning that the type of information stored is defined at the time of definition (when JSON data is created) and any errors will likely happen at the time of creation. It can easily store the following data types:
- text (strings)
- numbers
- objects
- arrays
- booleans (true or false)
- null value
Data format
The format of JSON data is readable by humans and its structure can contain the following six elements:
- beginning of an array, left square bracket “[“
- end of an array, right square bracket “]”
- beginning of an object, left curly bracket “{“
- end of an object, right curly bracket “}”
- name separator, colon “:”
- value separator “,”
JSON can contain any number of whitespace characters that could be automatically removed without affecting the meaning of the JSON data itself. These include space, tab, new line, carriage return (0x20, 0x09, 0x0a, 0x0d).
Text (strings) should be wrapped in quotation marks, both when used as names and values in JSON.
Objects are represented by a pair of curly brackets surrounding a pair of key / value pairs, similar to this :
{ "simpleobject": "hellovalue" }
{
"text1": "value1",
"text2": "value2",
"text3": "value3"
}
Arrays, on the other hand are simply represented by a list of values as follows:
[ "value1", "value2", "value3", ... ]
Values themselves can also be complex types so these can naturally be bested and mixed to describe complicated data relations, like this
{
"array1": [1, 2, 3, 4],
"string2": "hello",
"integer3": 445,
"intarray": [ 1, 5, 6, 7 ]
}
Opening a .json file
JSON formatted data files can be simply opened by any text editor and they have the “.json” extension. Even Notepad on Microsoft Windows can open them. Programmer’s editors offer better parsing and syntax highlighting so they should make it easier to work with.
For example on MacOS XCode (if installed) opens the file above similar to this:
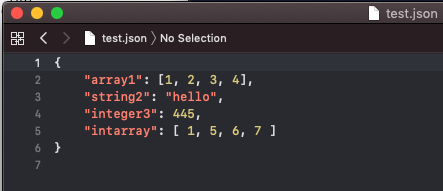
Using JSON data in JavaScript
Due to the fact that JSON was developed originally for JavaScript, it shares most of its syntax and features and it can be tempting to simply use “eval()” to evaluate and use JSON data. It mostly works OK but opens the code to various security and code injection vulnerabilities because new lines and special unicode characters are handled differently in JavaScript and JSON. Eval is also lacking error messages so it’s very difficult to debug data format problems, so the bottom line is: do not use eval() on JSON.
JavaScript provides special methods through its JSON object, to evaluate JSON data simply use
var testing = JSON.parse('{"array1": [1, 2, 3, 4], "string2": "hello", "integer3": 445, "intarray": [ 1, 5, 6, 7 ]}');
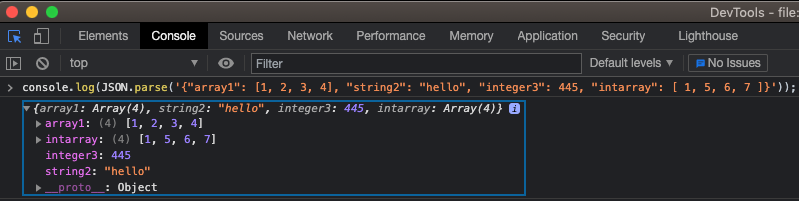
Its opposite, JSON.stringify() does the opposite, it takes any JavaScript variable as input and turns it into a JSON string representation. The example below also escaped quotes so it can directly be used in JavaScript.
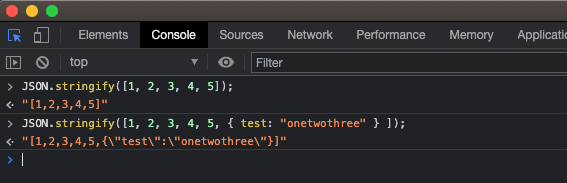
Using the example above, data elements can simply be referred as arrays or objects in JavaScript after parsing a JSON string so parts of our “testing” JS variable above can be accessed similary to any other JavaScript object or array
testing.array1 //the whole array containing [1,2,3,4]
testing.array1[3] // 4
testing.string2 // "hello"
Other uses
There is a complete API library that provides a standard way for web-based applications to communicate using JSON formatted data, called JSON API. Its documentation is available at https://jsonapi.org. On the client-side simple JavaScript is enough to support its functionality, although many different libraries exist to help with the implementation. On the server-side practically all server-side languages have working implementations, there is a quite extensive list at https://jsonapi.org/implementations/
Microsoft Excel can also use JSON formatted data sources using Power Query to import data using it as an external data source. The functionality is accessible on the Data ribbon or menu item then selecting Get Data / From file / From JSON.
JSON and XML comparison
While they offer similar functionality, there are some important differences between these two data formats. JSON has a lot simpler syntax and it’s easier for both computers and humans to parse and understand. The XML file format is a lot more verbose, too, because each opening tag should be closed with a matching tag and in the end, this redundancy adds a lot of extra information increasing the file size of XML documents. Because of the popularity of JavaScript, the availability of tools and the simplicity of the file format itself, JSON’s popularity quickly surpassed XML as shown by the amount of Google Trends searches :
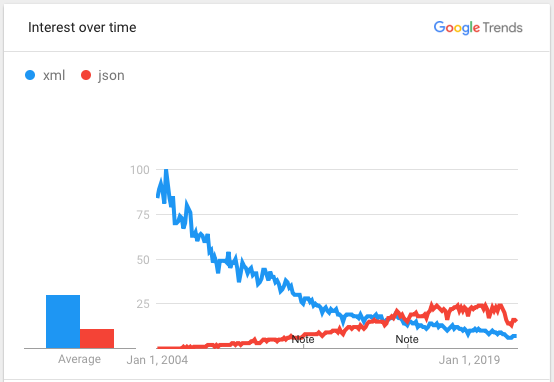
Parsing JSON is a matter of a function call in most languages and can be converted quickly and effectively into a ready-to-be-used data structure while XML take a lot more processing. Without conversion tools and pre-processing, XML data elements cannot be directly addressed unless each one is given a specific ID, and querying it involves learning a completely new programming language (XPath). Referencing a JSON formatted data element can simply be done just like any other array or object in the particular programming language.
Data format comparison – XML vs. JSON:
<developers>
<developer>
<name>Peter</name>
<language>JavaScript</language>
</developer>
<developer>
<name>Nancy</name>
<language>PHP</language>
</developer>
<developer>
<name>John</name>
<language>SQL</language>
</developer>
</developers>
{"developers": [
{"name": "Peter", "language": "JavaScript"},
{"name": "Nancy", "language": "PHP"},
{"name": "John", "language": "SQL"}
]}
XML is also weakly typed, meaning that the data definition itself (XML) doesn’t define the data type clearly in advance and they should be decided at the time of parsing. With JSON, data types are set at the time of definition, strings are in quotes, literals are either numbers or true / false / null values.